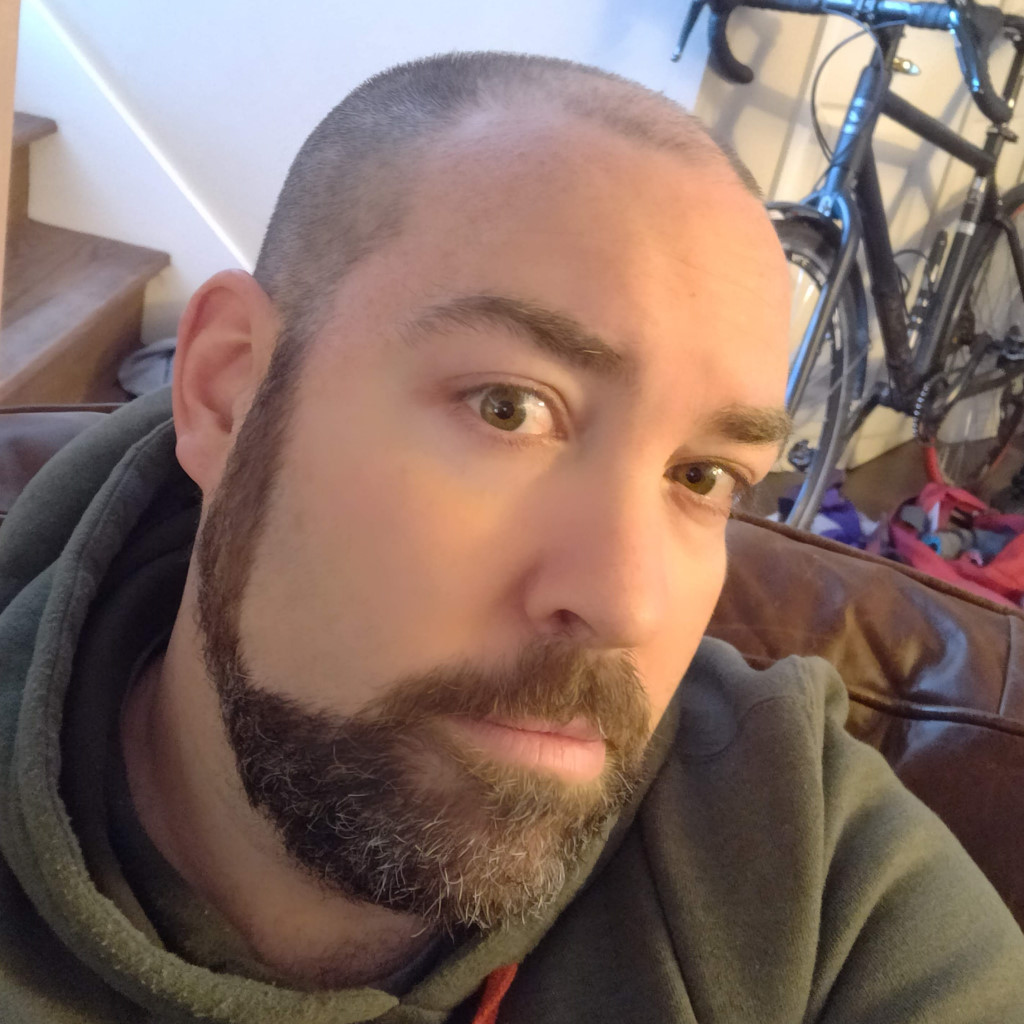
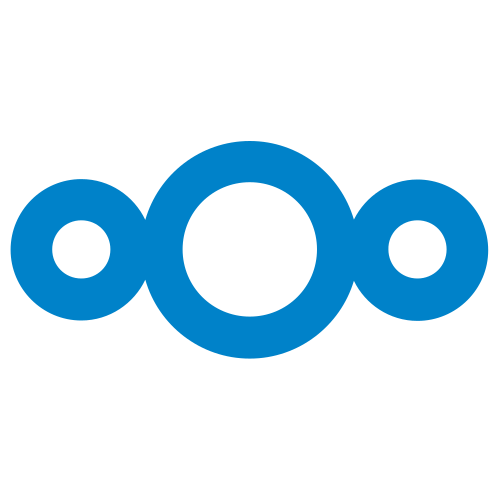
Hrm…I must have had something else wrong because I’m experiencing the same thing. I try to load a document that I created with the menu and it seems to time out. I thought that it was because of my Caddy config, but perhaps it wasn’t.
I only get: Document loading failed Failed to load Nextcloud Office - please try again later
The docker config is nothing special:
collabora: hostname: collabora image: collabora/code restart: "no" privileged: true cap_add: - MKNOD networks: - default env_file: collabora.env ports: - 8054:9980
Env: Besides
username
,password
, and this line:extra_params=--o:ssl.enable=true
I have 2 other variables set:
domain
andaliasgroup1
. Is domain supposed to refer to the Collabora domain or the NextCloud domain? I actually can’t remember where I got the aliasgroup1 from, but it was probably during my googling to try and get things working. I remember reading that the ‘.’ characters had to be escaped because those lines were used in a reflex.