
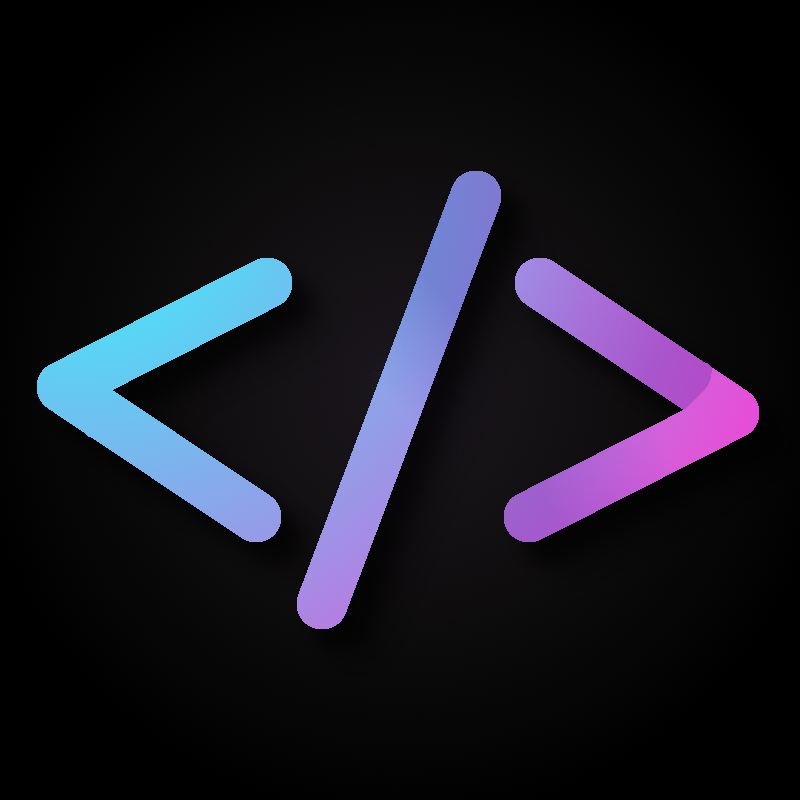
That’s how languages should all work?
While I agree, should be is not is. Also, Javascript is still a widely used and favored language, despite it’s flaws.
Sometimes people need to be convinced that there’s something better, hence all the articles of “Javascript devs discovering actual typing”, as you mentioned. Although it seems like the author already knew there’s better (I see Typescript and Rust on their Github), that they were just sharing Gleam.
As for specifically Gleam, I will say it’s a very nice language. Very simple to understand (with one minor exception: I personally find the use
keyword is a bit odd), strong typing, no collections of mysterious symbols (cough, Haskell), no metaprogramming, no lifetimes, no borrowing, no unclear polymorphism, no pointers, no nonsense. I like it, and am excited to see it grow
I find real-world examples help grasp concepts for me. I’ll use an example like a video platform (like Youtube) as an example for this:
One-to-one: for each one User, you maybe only allow one [content] Channel (or, could be one-or-none, meaning a User may have one Channel, or may use use their User account to be a viewer and have no Channel. You might decide to change this later to be one-to-many, but for the example let’s say you don’t). All the data could be stored just on the User entity, but maybe you decided to separate it into two tables so you don’t need to query the User entity which has sensitive info like a password, email, or physical address, when you just need a Channel. For each Channel, there is a foreign key pointing to the (owning_)user_id, and this is “unique”, so no duplicate id’s can exist in this column.
One-to-many (or Many-to-one): for each one Channel, there may be many Videos. You could use a relational table (channel_x_video), but this isn’t necessary. You can just store the (owning_)channel_id on the Video, and it will not be unique like the prior example, since a Channel will have many Videos, and many Videos belong to one Channel. The only real difference between “one-to-many” and “many-to-one” is semantic - the direction you look at it.
Many-to-many: many Users will watch many Videos. Maybe for saving whether a Video was watched by a User, you store it on a table (by the way, this example might not be the best example, but I was struggling to find a good example for many-to-many to fit the theme). Although each video-to-user relation may be one-to-one, many Videos will be watched by one User, and many Users will watch one Video. This would be not possible to store on both the Video or the User tables, so you will need a relational table (video_x_user, or include “watched” in the table name for clarity of purpose). The id column of each row is irrelevant, but each entry will need to store the video_id and the user_id (and neither will be unique), as well as maybe the percent watched as a float, the datetime it was watched, etc. Many-to-many relationships get very complicated often, so should be used carefully, especially when duplicate combinations of a_x_b can occur (here, we shouldn’t store a new row when a user watches a video a second time)